In this article, we'll be looking at the newly added NestJS CLI Resource Generators, and how they can greatly speed up the development process by generating all the files and boilerplate code required for CRUD applications.
In case you're not familiar with NestJS, it is a TypeScript Node.js framework that helps you build enterprise-grade efficient and scalable Node.js applications.
Introduction to CLI Generators
Throughout the life span of a project, when we build new features, we often need to add new resources to our application. These resources typically require multiple, repetitive operations that we have to repeat each time we define a new resource.
Let's imagine a real-world scenario, where we need to expose CRUD endpoints for 2 entities, let's say User and Product entities. Following the best practices, for each entity we would have to perform several operations, as follows:
- Generate a module (
nest g mo
) to keep code organized and establish clear boundaries (grouping related components) - Generate a controller (
nest g co
) to define CRUD routes (or queries/mutations for GraphQL applications) - Generate a service (
nest g s
) to implement & isolate business logic - Generate an entity class/interface to represent the resource data shape
- Generate Data Transfer Objects (or inputs for GraphQL applications) to define how the data will be sent over the network
That's a lot of steps!
To help speed up this repetitive process, NestJS CLI now provides a new generator (schematic) that automatically generates all the boilerplate code to help us avoid doing all of this, and make the developer experience much simpler.
Resource Generator schematic supports generating HTTP controllers, Microservice controllers, GraphQL resolvers (both code first and schema first), and WebSocket Gateways.
Generating a CRUD API
Hint Before you proceed, make sure to install the latest version of
@nestjs/cli
and@nestjs/schematics
packages.
To create a new resource, simply run the following command in the root directory of your project:
$ nest g resource
The CLI will prompt you with a few questions, as shown on the animation below:
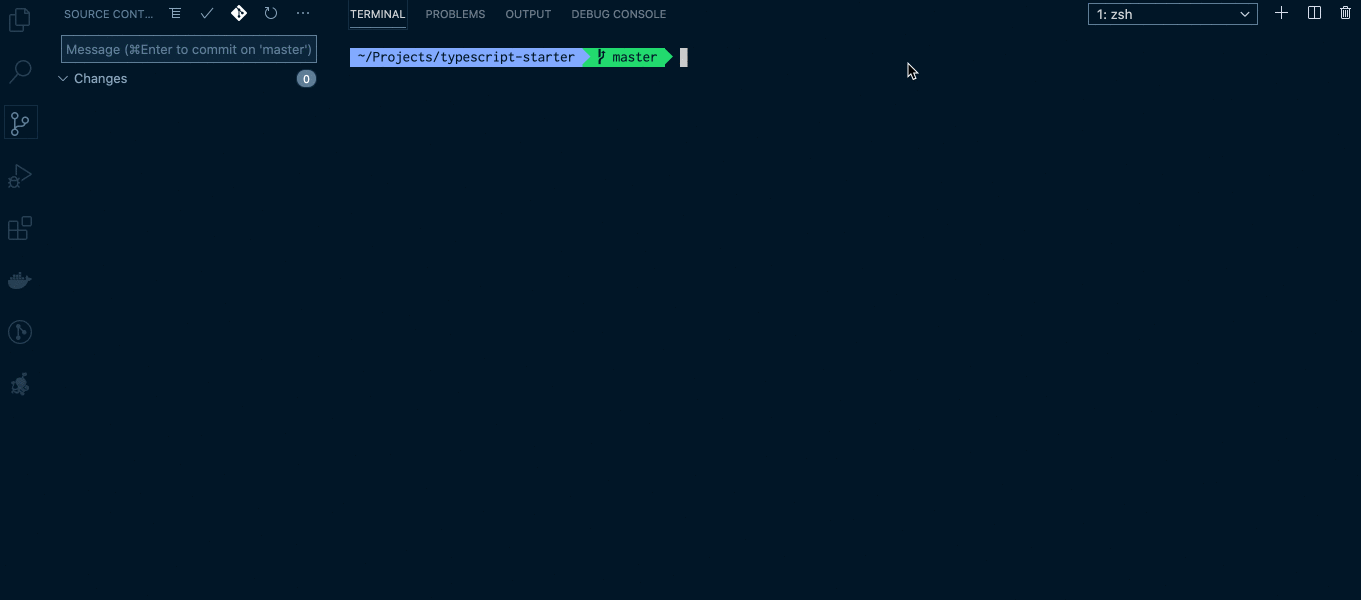
As you can see on the example above, nest g resource
command not only generates all the NestJS building blocks (module, service, controller classes) but also an entity class, DTO classes as well as the testing (.spec
) files.
Also, it automatically creates placeholders for all the CRUD endpoints (routes for REST APIs, queries and mutations for GraphQL, message subscribes for both Microservices and WebSocket Gateways) - all without having to lift a finger!
NOTE: Generated service classes are not tied to any specific ORM (or data source). This makes the generator generic enough to meet the needs of any project. By default, all methods will contain placeholders, allowing you to populate it with the data sources specific to your project.
Likewise, if you want to generate resolvers for a GraphQL application, simply select the GraphQL (code first)
(or GraphQL (schema first)
) as your transport layer.
In this case, NestJS will generate a resolver class instead of a REST API controller:
$ nest g resource users> ? What transport layer do you use? GraphQL (code first)> ? Would you like to generate CRUD entry points? Yes> CREATE src/users/users.module.ts (224 bytes)> CREATE src/users/users.resolver.spec.ts (525 bytes)> CREATE src/users/users.resolver.ts (1109 bytes)> CREATE src/users/users.service.spec.ts (453 bytes)> CREATE src/users/users.service.ts (625 bytes)> CREATE src/users/dto/create-user.input.ts (195 bytes)> CREATE src/users/dto/update-user.input.ts (281 bytes)> CREATE src/users/entities/user.entity.ts (187 bytes)> UPDATE src/app.module.ts (312 bytes)
Hint To avoid generating test files, you can pass the
--no-spec
flag, as follows:nest g resource users --no-spec
Let's look at the code generated above for the UsersResolver
class.
We can see below, that not only were all boilerplate mutations and queries created, but everything is all tied together. We're utilizing the UsersService
, User
Entity, and even our DTO's!
import { Resolver, Query, Mutation, Args, Int } from '@nestjs/graphql';import { UsersService } from './users.service';import { User } from './entities/user.entity';import { CreateUserInput } from './dto/create-user.input';import { UpdateUserInput } from './dto/update-user.input';@Resolver(() => User)export class UsersResolver { constructor(private readonly usersService: UsersService) {} @Mutation(() => User) createUser(@Args('createUserInput') createUserInput: CreateUserInput) { return this.usersService.create(createUserInput); } @Query(() => [User], { name: 'users' }) findAll() { return this.usersService.findAll(); } @Query(() => User, { name: 'user' }) findOne(@Args('id', { type: () => Int }) id: number) { return this.usersService.findOne(id); } @Mutation(() => User) updateUser(@Args('updateUserInput') updateUserInput: UpdateUserInput) { return this.usersService.update(updateUserInput.id, updateUserInput); } @Mutation(() => User) removeUser(@Args('id', { type: () => Int }) id: number) { return this.usersService.remove(id); }}
In Conclusion
We hope that the new NestJS resource
schematic helps speed up and improve the developer experience tremendously, letting us build our Nest applications that much faster.
Remember, Nest is open source, and if you have any amazing ideas to improve the schematic, PRs are always welcome!
Official NestJS Courses 📚
Looking to level-up your NestJS and Node.js ecosystem skills?
The NestJS Fundamentals Course is now LIVE and 25% off for a limited time!
In this workshop-style course, we'll incrementally build a NestJS application, learning all of the fundamental building blocks, tips & tricks, and best-practices along the way!
Support
Nest is an MIT-licensed open source project with its ongoing development made possible thanks to the support by the community, thank you!
<script>We strongly believe that someday we could fully focus on #opensource to make @nodejs world better 🚀🔥 @opencollect
— NestJS (@nestframework) April 25, 2018
- enjoy using @nestframework?
- ask your company to support us:
- https://t.co/taYS49lllr pic.twitter.com/L1O9Vf5uhS
If you want to join them, you can find more here.
Official consulting
Our goal is to help elevate teams — giving them the push they need to truly succeed in today’s ever-changing tech world. Contact us to find out more about expertise consulting, code reviews, architectural support, on-site enterprise workshops, trainings, and private sessions: support@nestjs.com.
Thank you
To all backers, sponsors, contributors, and community, thank you once again! This product is for you. And this is only the beginning of the long 🚀 story.
Become a Backer or Sponsor to Nest by donating to our open collective. ❤
Learn NestJS - Official NestJS Courses 📚
Level-up your NestJS and Node.js ecosystem skills in these incremental workshop-style courses, from the NestJS Creator himself, and help support the NestJS framework! 🐈🚀 The NestJS Fundamentals Course is now LIVE and 25% off for a limited time!
🎉 NEW - NestJS Course Extensions now live!
- NestJS Advanced Concepts Course now LIVE!
- NestJS Advanced Bundle (Advanced Architecture and Advanced Concepts) now 22% OFF!
- NestJS Microservices now LIVE!
- NestJS Authentication / Authorization Course now LIVE!
- NestJS GraphQL Course (code-first & schema-first approaches) are now LIVE!
- NestJS Authentication / Authorization Course now LIVE!